As blockchain computing continues to steadily gain momentum across various industries, relevant platforms such as Ethereum, Hyperledger, etc, have emerged and prospered. Even though the term blockchain has evolved beyond a mere keyword for cryptocurrency, its core underlying operational structure still adheres to how a cryptocurrency fundamentally maintains a decentralized ledger — that is, as distributed copies of a growing blockchain kept by individual nodes on the system to agree on an eventual version of the blockchain via a consensual algorithm.
Blockchain application using Akka classic actors
In 2020, I developed an Actor-based blockchain application (source code at GitHub) in Scala using Akka Actor’s classic API. While it’s primarily for proof of concept, the application does utilize relevant cryptographic functions (e.g. public key cryptography standards), hash data structure (e.g. Merkle trees), along with a simplified proof-of-work consensus algorithm to simulate mining of a decentralized cryptocurrency on a scalable cluster.
Since the blockchain application consists of just a handful of Actors mostly handling common use cases, migrating it to using the typed Actor API serves a great trial exercise for something new. While it was never expected to be a trivial find-and-replace task, it was also not a particularly difficult one. A recent mini-blog series highlights the migration how-to’s of some key actor/cluster features used by the blockchain application.
The Akka Typed blockchain application
For the impatient, source code for the Akka Typed blockchain application is at this GitHub link.
Written in Scala, the build tool for the application is the good old sbt
, with the library dependencies specified in “{project-root}/built.sbt”. Besides akka-actor-typed
and akka-cluster-typed
for the typed actor/cluster features, Bouncy Castle and Apache Commons Codec are included for processing public key files in PKCS#8 PEM format.
For proof of concept purpose, the blockchain application can be run on a single computer on multiple Shell command terminals, using the default configurations specified in “{project-root}/src/main/resources/application.conf”. The configuration file consists of information related to Akka cluster/remoting transport protocol, seed nodes, etc. The cluster setup can be reconfigured to run on an actual network of computer nodes in the cloud. Also included in the configuration file are a number of configurative parameters for mining of the blockchain, such as mining reward, time limit, proof-of-work difficulty level, etc.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
akka { actor { provider = "cluster" default-dispatcher { throughput = 10 } allow-java-serialization = on warn-about-java-serializer-usage = off } remote.artery { enabled = on transport = tcp canonical { hostname = "127.0.0.1" port = 2551 } } cluster { seed-nodes = [ "akka://blockchain@127.0.0.1:2551", "akka://blockchain@127.0.0.1:2552" ] } } blockchain { proof-of-work { difficulty = 3 nonce = 0 reward = 99 network-name = "akka-blockchain" network-account-key = "MIICIjANBgkqhkiG9w0BAQEFAAOCAg8AMIICCgKCAgEApKyCqvNjx+YfVYFippxviV0UgEgpYJbV0luEJTkcbnvIIolqXR5JJACbk8TDW72F9pnwKqQhM0vzpsbat+kubl5FeO0CPk/Gk2/aAcoP11DNqYAgqvCKEyZlJFmwnC/6JecCBdBb+G/+v894LESD8y4upjZIRdrrC4UQowlm4+6mPJTbB0U+2q7rwv8FXmR9KHmRxtGoMh885bKTXwqOO0pqh0/MMSaW5pzS6s5bUiX7ekl0EPwTyiXbuyUZ3nZcpm/v7lCvXWlyRju8g2pg9e0BShyfI7w1qqw1xEeRVwU1qIlN4Q1bz82t1O+7l308VtiXH2lM9Vhn+Sfz68cjtmJ4uSwZKeV91kaOJdr1OafM9ryfPv2MGDVvSGUk+TEMORysFcRS59VsON8styFJ6hGx1/GKyfUilCS2G2dS/9NvTJjV64nPTQ1mufT4Kd8tBa3/DTmjrqsFjfzYsc/kVz0QsGN7PxgKPf5+aSzrG2y9GQy69jAb6wpMoLcux2zlZvobYR+qjoBIsn+5Y5J0Fs9Jvwh0yd0KGdaDkMZnPGYTQo1vpC4gqowt7q4lba0FRV3jt/bm1B8Pu+YWk4MasNk1wfBMLNOr1CGaiSXFIl0NyATViOOyq6FEnHdF8L1MmLM9v7Yj3l4q5ykF0u/JnDXErC7CwtECRVr6gX3LWfcCAwEAAQ==" } timeout { mining = 20000 block-validation = 1000 } simulator { transaction-feed-interval = 15000 mining-average-interval = 20000 } } |
The underlying data structures of the blockchain
Since all the changes to the blockchain application are only for migrating actors to Akka Typed, the underlying data structures for the blockchain, its inner structural dependencies as well as associated cryptographic functions remain unchanged.
As an attempt to make this blog post an independent one, some content of the application overview is going to overlap the previous overview for the Akka classic application. Nonetheless, I’m including some extra diagrams for a little more clarity.
Below is a diagram showing the blockchain’s underlying data structures:
- Account, TransactionItem, Transactions
- MerkleTree
- Block, RootBlock, LinkedBlock
- ProofOfWork
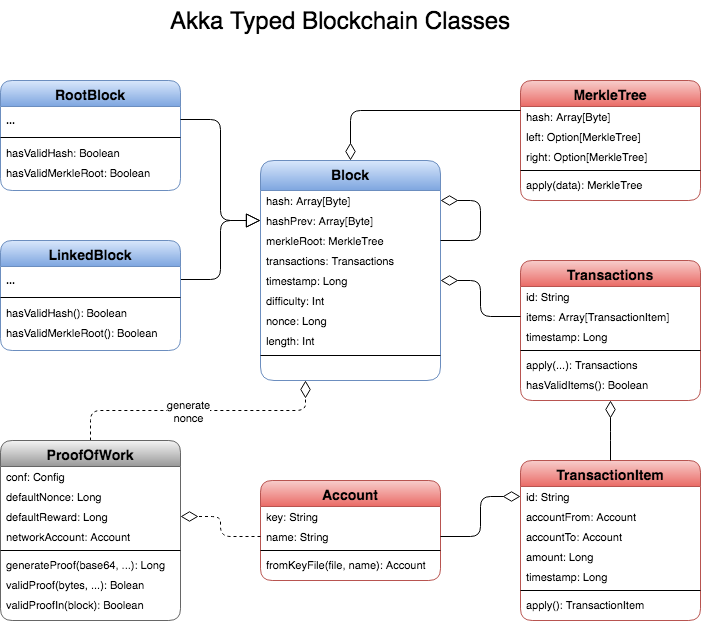
The centerpiece of the blockchain data structures is the abstract class Block
which is extended by RootBlock
(i.e. the “genesis block”) and LinkedBlock
. Each of the individual blocks is identified by the hash
field and backward-linked to its predecessor via hashPrev
.
Field difficulty
carries the difficulty level pre-set in the application configuration. The nonce
field is initialized also from configuration and will be updated with the proof
value returned from the proof-of-work consensual algorithm (which is difficulty-dependent).
Class Transactions
represents a sequence of transaction items, along with the sender/receiver (of type Account
) and timestamp. Both the transaction sequence and its hashed value merkleRoot
are kept in a Block
object. The Account
class is identified by field key
which is the Base64 public key of the PKCS keypair possessed by the account owner. As for object ProofOfWork
, it’s a “static” class for keeping the consensual algorithmic methods for proof-of-work.
For a deeper dive of the various objects’ inner workings, please read the following blogs:
Source code for the data structures can be found under akkablockchain/model
in the GitHub repo.
The typed actors that “run” the blockchain
As for the actors, aside from being revised from loosely to strictly typed actors, their respective functionalities within a given cluster node as well as among the peer actors on other cluster nodes remain unchanged.
The following diagram highlights the hierarchical flow logic of the various actors on a given cluster node:
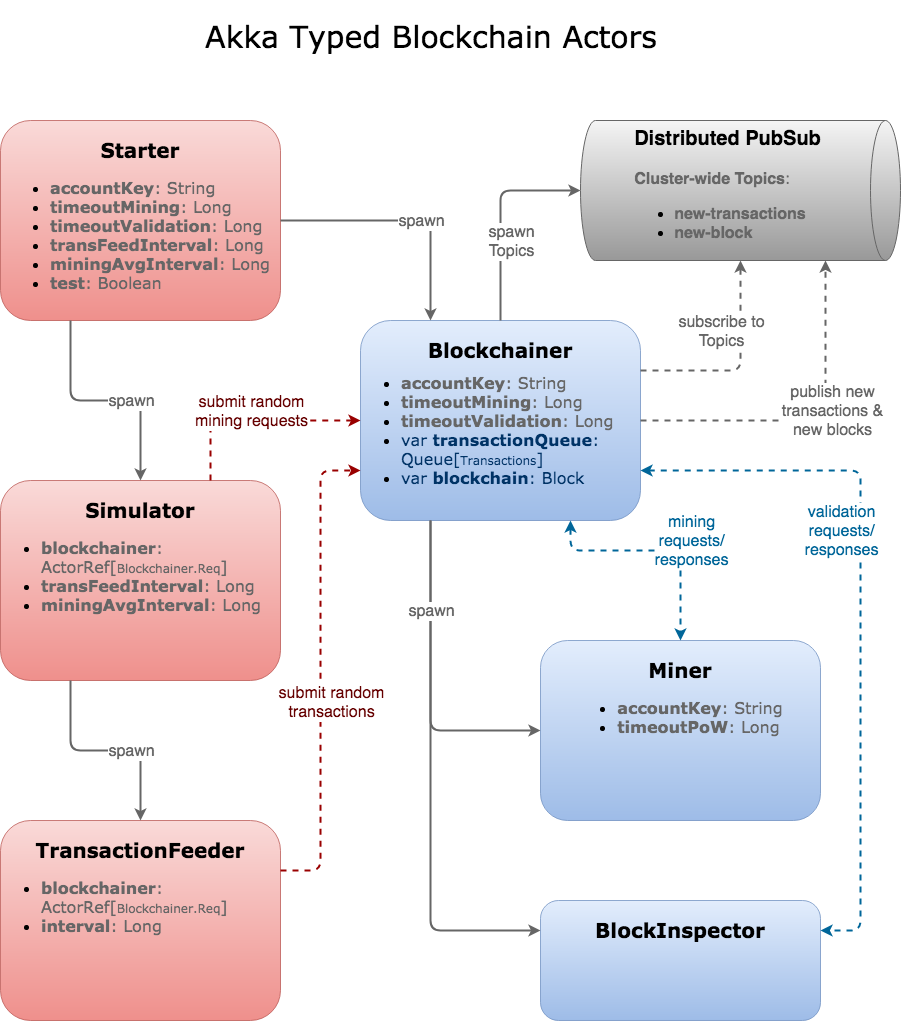
Starter – On each cluster node, the main
program of blockchain application is initialized with starting up the top-level Starter
actor (a.k.a. the user guardian
), which in turn spawns two actors: Blockchainer
and Simulator
.
Blockchainer – The Blockchainer
actor on any given cluster node maintains a copy of the blockchain and transaction queue for a miner
identified by their cryptographic public key. It collects submitted transactions in the queue and updates the blockchain according to the proof-of-work consensual rules by means of the cluster-wide distributed pub/sub. The actor delegates mining work to its child actor Miner
and validation of mined blocks to child actor BlockInspector
.
Miner – The mining tasks of carrying out computationally demanding proof-of-work is handled by the Miner
actor. Using an asynchronous routine bound by a configurable time-out, actor Miner
returns the proofs back to the parent actor via the Akka request-response ask
queries.
BlockInspector – This other child actor of Blockchainer
is responsible for validating content of a newly mined block before it can be appended to the existing blockchain. The validation verifies that generated proof within the block as well as the intertwined hash values up the chain of the historical blocks. The result is then returned to the parent actor via Akka ask
.
Simulator – Actor Simulator
simulates mining requests and transaction submissions sent to the Blockchainer
actor on the same node. It generates periodic mining requests by successively calling Akka scheduler function scheduleOnce with random variations of configurable time intervals. Transaction submissions are delegated to actor TransactionFeeder
.
TransactionFeeder – This child actor of Simulator
periodically submits transactions to actor Blockchainer via an Akka scheduler. Transactions are created with random user accounts and transaction amounts. Accounts are represented by their cryptographic public keys. For demonstration purpose, a number of PKCS#8 PEM keypair files were created and kept under “{project-root}/src/main/resources/keys/” to save initial setup time.
Since the overall functional flow of this application remains the same as the old one, this previously published diagram is also worth noting:
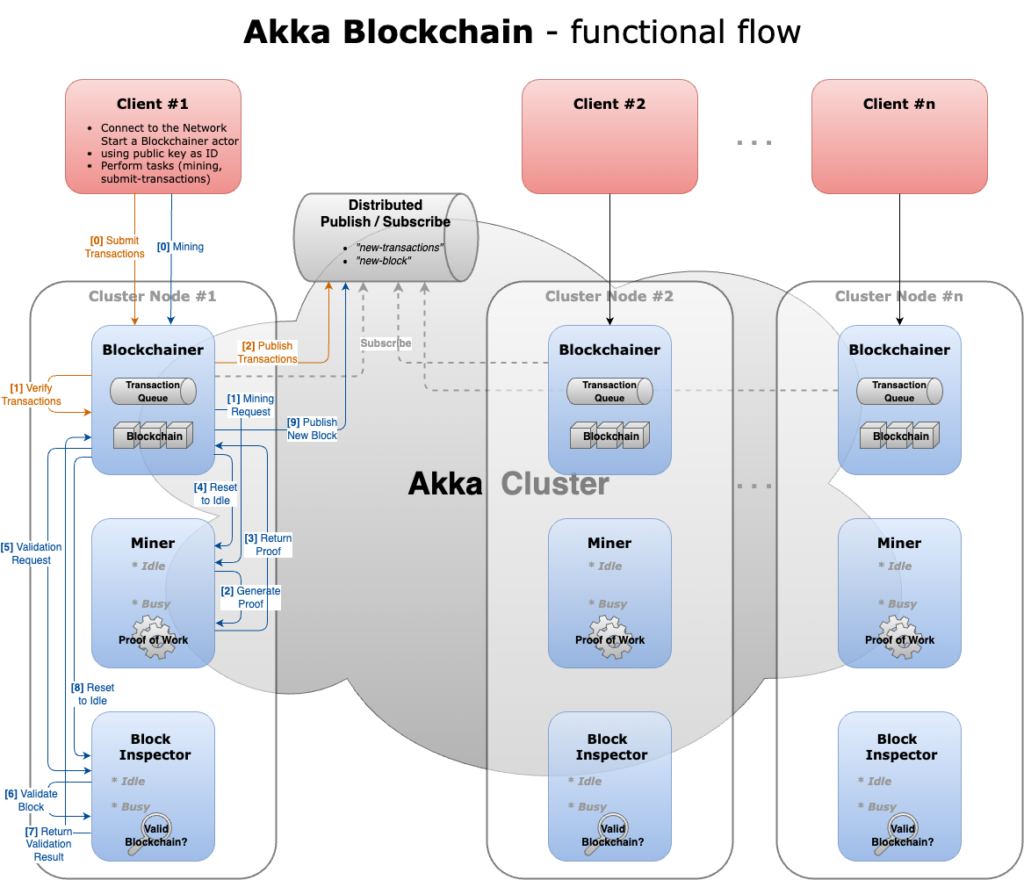
Source code for the actors can be found under akkablockchain/actor
in the GitHub repo.
Areas that could be feature-enhanced
This blockchain application is primarily for proof of concept, thus the underlying data structure and security features have been vastly simplified. For it to get a little closer to a real-world cryptocurrency, addition/enhancement of features in a few areas should be considered. The following bullet items are a re-cap of the “Feature enhancement” section from the old blog:
Data encryption: The transactions stored in the blockchain unencrypted. Individual transaction items could be encrypted, each of which to be stored with the associated cryptographic signature, requiring miners to verify the signature while allowing only those who have the corresponding private key for the transaction items to see the content.
Self regulation: A self-regulatory mechanism that adjusts the difficulty level of the Proof-of-Work in accordance with network load would help stabilize the digital currency. For example, in the event of a significant plunge, Bitcoin would impose self-regulatory reduction in the PoW difficulty requirement to temporarily make mining more rewarding that helped dampen the fall.
Currency supply: In a cryptocurrency like Bitcoin, issuance of the mining reward by the network is essentially how the digital coins are “minted”. To keep inflation rate under control as the currency supply increases, the rate of coin minting must be proportionately regulated over time. Bitcoin has a periodic “halfing” mechanism that reduces the mining reward by half for every 210,000 blocks added to the blockchain and will cease producing new coins once the total supply reaches 21 million coins.
Blockchain versioning: Versioning of the blockchain would make it possible for future feature enhancement, algorithmic changes or security fix by means of a fork
, akin to Bitcoin’s soft/hard forks
, without having to discard the old system.
User Interface: The existing application focuses mainly on how to operate a blockchain network, thus supplementing it with, say, a Web-based user interface (e.g. using Akka HTTP/Play framework) for miners to participate mining would certainly make it a more user-friendly system.
Sample console log: running on 3 cluster nodes
Running the application and examining its output from the console log would reveal how multiple miners, each on a separate cluster node, “collaboratively” compete for growing a blockchain with a consensual algorithm. In particular, pay attention to:
- attempts by individual miners to succeed or fail in building new blocks due to timeout by the Proof-of-Work routine
- miners’ requests for adding new blocks to their blockchain rejected by their occupied mining actor
- how the individual copies of the blockchain possessed by the miners “evolve” by accepting the first successfully expanded blockchain done by themselves or their peers
- the number of trials in Proof-of-Work for a given block (i.e. displayed as
BLK()
) as its rightmost argument (e.g.BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128)
; for more details about how the mining of blockchain works, please see this blog post) - how Akka cluster nodes switch their “leader” upon detecting failure (due to termination by users, network crashes, etc)
Below is sample output of running the blockchain application with default configuration on 3 cluster nodes.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 |
### Console Log: CLUSTER NODE #1 $ sbt "runMain akkablockchain.Main 2551 src/main/resources/keys/account0_public.pem" [info] running akkablockchain.Main 2551 src/main/resources/keys/account0_public.pem 11:04:00.948 [blockchain-akka.actor.default-dispatcher-3] INFO akka.event.slf4j.Slf4jLogger - Slf4jLogger started SLF4J: A number (1) of logging calls during the initialization phase have been intercepted and are SLF4J: now being replayed. These are subject to the filtering rules of the underlying logging system. SLF4J: See also http://www.slf4j.org/codes.html#replay 11:04:01.309 [blockchain-akka.actor.default-dispatcher-3] INFO akka.remote.artery.tcp.ArteryTcpTransport - Remoting started with transport [Artery tcp]; listening on address [akka://blockchain@127.0.0.1:2551] with UID [5401420401358518049] 11:04:01.337 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Starting up, Akka version [2.6.13] ... 11:04:01.461 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Registered cluster JMX MBean [akka:type=Cluster] 11:04:01.462 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Started up successfully 11:04:01.486 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - No downing-provider-class configured, manual cluster downing required, see https://doc.akka.io/docs/akka/current/typed/cluster.html#downing 11:04:01.831 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] idle: Got akka.actor.LightArrayRevolverScheduler$TaskHolder@540fe169 11:04:01.833 [blockchain-akka.actor.default-dispatcher-3] DEBUG akka.actor.typed.pubsub.Topic$ - Starting up pub-sub topic [new-transactions] for messages of type [akkablockchain.actor.Blockchainer$Req] 11:04:01.836 [blockchain-akka.actor.default-dispatcher-17] DEBUG akka.actor.typed.pubsub.Topic$ - Starting up pub-sub topic [new-block] for messages of type [akkablockchain.actor.Blockchainer$Req] 11:04:01.848 [blockchain-akka.actor.default-dispatcher-17] DEBUG akka.actor.typed.pubsub.Topic$ - Local subscriber [Actor[akka://blockchain/user/blockchainer#715764424]] added, went from no subscribers to one, subscribing to receptionist 11:04:01.848 [blockchain-akka.actor.default-dispatcher-3] DEBUG akka.actor.typed.pubsub.Topic$ - Local subscriber [Actor[akka://blockchain/user/blockchainer#715764424]] added, went from no subscribers to one, subscribing to receptionis 11:04:01.879 [blockchain-akka.actor.internal-dispatcher-13] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2551] - Actor was registered: [ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block)] [akka://blockchain/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049] 11:04:01.882 [blockchain-akka.actor.default-dispatcher-15] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set()] 11:04:01.883 [blockchain-akka.actor.internal-dispatcher-13] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2551] - Actor was registered: [ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions)] [akka://blockchain/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049] 11:04:01.884 [blockchain-akka.actor.default-dispatcher-3] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set()] 11:04:01.957 [blockchain-akka.actor.default-dispatcher-3] WARN akka.stream.Materializer - [outbound connection to [akka://blockchain@127.0.0.1:2552], control stream] Upstream failed, cause: StreamTcpException: Tcp command [Connect(127.0.0.1:2552,None,List(),Some(5000 milliseconds),true)] failed because of java.net.ConnectException: Connection refused 11:04:01.958 [blockchain-akka.actor.default-dispatcher-3] WARN akka.stream.Materializer - [outbound connection to [akka://blockchain@127.0.0.1:2552], message stream] Upstream failed, cause: StreamTcpException: Tcp command [Connect(127.0.0.1:2552,None,List(),Some(5000 milliseconds),true)] failed because of java.net.ConnectException: Connection refused 11:04:02.382 [blockchain-akka.actor.internal-dispatcher-13] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2551] - Change from replicator: [Map(ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block) -> Set(akka://blockchain/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049), ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions) -> Set(akka://blockchain/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049))], changes: [(new-block,[akka://blockchain/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049]), (new-transactions,[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049])], tombstones [] 11:04:02.385 [blockchain-akka.actor.default-dispatcher-15] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2092003166])] 11:04:02.385 [blockchain-akka.actor.default-dispatcher-3] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#-497024677])] 11:04:02.860 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(5c75, 4500/2, 2021-05-11 18:04:02) to Blockchainer. 11:04:02.865 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@7d1a9568: T(5c75, 4500/2, 2021-05-11 18:04:02) is published. 11:04:02.869 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(5c75, 4500/2, 2021-05-11 18:04:02) to transaction queue. 11:04:06.603 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Node [akka://blockchain@127.0.0.1:2551] is JOINING itself (with roles [dc-default], version [0.0.0]) and forming new cluster 11:04:06.604 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - is the new leader among reachable nodes (more leaders may exist) 11:04:06.611 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Leader is moving node [akka://blockchain@127.0.0.1:2551] to [Up] <<< <<<--- Cluster seed node #1, bound to port# 2551, is up <<< 11:04:17.853 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(fab1, 3000/1, 2021-05-11 18:04:17) to Blockchainer. 11:04:17.854 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@7d1a9568: T(fab1, 3000/1, 2021-05-11 18:04:17) is published. 11:04:17.855 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(fab1, 3000/1, 2021-05-11 18:04:17) to transaction queue. 11:04:21.638 [blockchain-akka.actor.default-dispatcher-3] WARN akka.remote.artery.Association - Outbound control stream to [akka://blockchain@127.0.0.1:2552] failed. Restarting it. akka.remote.artery.OutboundHandshake$HandshakeTimeoutException: Handshake with [akka://blockchain@127.0.0.1:2552] did not complete within 20000 ms 11:04:31.870 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 16000 millis 11:04:31.870 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:04:31.872 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(5c75, 4500/2, 2021-05-11 18:04:02), T(fab1, 3000/1, 2021-05-11 18:04:17)) 11:04:31.875 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:04:32.859 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(5f17, 2000/1, 2021-05-11 18:04:32) to Blockchainer. 11:04:32.860 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@7d1a9568: T(5f17, 2000/1, 2021-05-11 18:04:32) is published. 11:04:32.861 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(5f17, 2000/1, 2021-05-11 18:04:32) to transaction queue. 11:04:47.867 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(9462, 7000/3, 2021-05-11 18:04:47) to Blockchainer. 11:04:47.868 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@7d1a9568: T(9462, 7000/3, 2021-05-11 18:04:47) is published. 11:04:47.868 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(9462, 7000/3, 2021-05-11 18:04:47) to transaction queue. 11:04:47.922 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 26000 millis 11:04:47.923 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:04:47.925 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(fab1, 3000/1, 2021-05-11 18:04:17), T(5f17, 2000/1, 2021-05-11 18:04:32), T(9462, 7000/3, 2021-05-11 18:04:47)) 11:04:47.925 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:05:00.185 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:05:00.191 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@7d1a9568: BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435) is valid. Updating blockchain. 11:05:00.192 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:05:00.463 [blockchain-akka.actor.default-dispatcher-18] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Received InitJoin message from [Actor[akka://blockchain@127.0.0.1:2552/system/cluster/core/daemon/joinSeedNodeProcess-1#-1847203672]] to [akka://blockchain@127.0.0.1:2551] 11:05:00.463 [blockchain-akka.actor.default-dispatcher-18] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Sending InitJoinAck message from node [akka://blockchain@127.0.0.1:2551] to [Actor[akka://blockchain@127.0.0.1:2552/system/cluster/core/daemon/joinSeedNodeProcess-1#-1847203672]] (version [2.6.13]) 11:05:00.566 [blockchain-akka.actor.default-dispatcher-18] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Node [akka://blockchain@127.0.0.1:2552] is JOINING, roles [dc-default], version [0.0.0] 11:05:00.569 [blockchain-akka.actor.internal-dispatcher-4] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2551] - Node added [UniqueAddress(akka://blockchain@127.0.0.1:2552,-3750460307660254480)] 11:05:00.658 [blockchain-akka.actor.default-dispatcher-18] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Leader is moving node [akka://blockchain@127.0.0.1:2552] to [Up] <<< <<<--- Seed node #2, bound to port# 2552, joined the cluster <<< 11:05:01.159 [blockchain-akka.actor.internal-dispatcher-12] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2551] - Change from replicator: [Map(ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions) -> Set(akka://blockchain/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480), ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block) -> Set(akka://blockchain/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480))], changes: [(new-block,[akka://blockchain/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480]), (new-transactions,[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480])], tombstones [] 11:05:01.160 [blockchain-akka.actor.default-dispatcher-17] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#-497024677], Actor[akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180])] 11:05:01.160 [blockchain-akka.actor.default-dispatcher-18] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2092003166], Actor[akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065])] 11:05:02.871 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(ac20, 2500/1, 2021-05-11 18:05:02) to Blockchainer. 11:05:02.871 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@7d1a9568: T(ac20, 2500/1, 2021-05-11 18:05:02) is published. 11:05:02.872 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(ac20, 2500/1, 2021-05-11 18:05:02) to transaction queue. 11:05:03.284 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(7634, 5000/3, 2021-05-11 18:05:03) to transaction queue. 11:05:13.981 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 25000 millis 11:05:13.981 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:05:13.982 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(5f17, 2000/1, 2021-05-11 18:04:32), T(9462, 7000/3, 2021-05-11 18:04:47), T(ac20, 2500/1, 2021-05-11 18:05:02), T(7634, 5000/3, 2021-05-11 18:05:03)) 11:05:13.982 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:05:15.739 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:05:15.741 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@7d1a9568: BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128) is valid. Updating blockchain. 11:05:15.741 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:05:17.871 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(a545, 2500/1, 2021-05-11 18:05:17) to Blockchainer. 11:05:17.872 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@7d1a9568: T(a545, 2500/1, 2021-05-11 18:05:17) is published. 11:05:17.872 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(a545, 2500/1, 2021-05-11 18:05:17) to transaction queue. 11:05:18.265 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(6e8e, 6500/3, 2021-05-11 18:05:18) to transaction queue. 11:05:32.879 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(862f, 1000/1, 2021-05-11 18:05:32) to Blockchainer. 11:05:32.880 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@7d1a9568: T(862f, 1000/1, 2021-05-11 18:05:32) is published. 11:05:32.880 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(862f, 1000/1, 2021-05-11 18:05:32) to transaction queue. 11:05:33.285 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(a81d, 7000/3, 2021-05-11 18:05:33) to transaction queue. 11:05:39.038 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 25000 millis 11:05:39.038 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:05:39.040 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(9462, 7000/3, 2021-05-11 18:04:47), T(ac20, 2500/1, 2021-05-11 18:05:02), T(7634, 5000/3, 2021-05-11 18:05:03), T(a545, 2500/1, 2021-05-11 18:05:17), T(6e8e, 6500/3, 2021-05-11 18:05:18), T(862f, 1000/1, 2021-05-11 18:05:32), T(a81d, 7000/3, 2021-05-11 18:05:33)) 11:05:39.041 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:05:41.914 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:05:41.916 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@7d1a9568: BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242) is valid. Updating blockchain. 11:05:41.917 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:05:44.516 [blockchain-akka.actor.default-dispatcher-5] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Received InitJoin message from [Actor[akka://blockchain@127.0.0.1:2553/system/cluster/core/daemon/joinSeedNodeProcess-1#-236485565]] to [akka://blockchain@127.0.0.1:2551] 11:05:44.516 [blockchain-akka.actor.default-dispatcher-5] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Sending InitJoinAck message from node [akka://blockchain@127.0.0.1:2551] to [Actor[akka://blockchain@127.0.0.1:2553/system/cluster/core/daemon/joinSeedNodeProcess-1#-236485565]] (version [2.6.13]) 11:05:44.603 [blockchain-akka.actor.default-dispatcher-5] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Node [akka://blockchain@127.0.0.1:2553] is JOINING, roles [dc-default], version [0.0.0] 11:05:44.604 [blockchain-akka.actor.internal-dispatcher-20] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2551] - Node added [UniqueAddress(akka://blockchain@127.0.0.1:2553,5558056305511553349)] 11:05:45.372 [blockchain-akka.actor.internal-dispatcher-7] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2551] - Change from replicator: [Map(ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block) -> Set(akka://blockchain/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480, akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349), ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions) -> Set(akka://blockchain/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480, akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349))], changes: [(new-transactions,[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480, akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349]), (new-block,[akka://blockchain/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480, akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349])], tombstones [] 11:05:45.373 [blockchain-akka.actor.default-dispatcher-5] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2092003166], Actor[akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065], Actor[akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewTransactions#-2035531976])] 11:05:45.374 [blockchain-akka.actor.default-dispatcher-5] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#-497024677], Actor[akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180], Actor[akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewBlock#1714452195])] 11:05:45.550 [blockchain-akka.actor.default-dispatcher-5] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Leader is moving node [akka://blockchain@127.0.0.1:2553] to [Up] <<< <<<--- Node #3, bound to port# 2553, joined the cluster <<< 11:05:47.394 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(69d4, 4500/2, 2021-05-11 18:05:47) to transaction queue. 11:05:47.882 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(0060, 2000/1, 2021-05-11 18:05:47) to Blockchainer. 11:05:47.882 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@7d1a9568: T(0060, 2000/1, 2021-05-11 18:05:47) is published. 11:05:47.883 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(0060, 2000/1, 2021-05-11 18:05:47) to transaction queue. 11:05:48.284 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(bca5, 4000/3, 2021-05-11 18:05:48) to transaction queue. 11:05:53.530 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@7d1a9568: BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567) is valid. Updating blockchain. 11:05:53.530 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:02.371 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(b137, 3500/2, 2021-05-11 18:06:02) to transaction queue. 11:06:02.887 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(3b6c, 5500/2, 2021-05-11 18:06:02) to Blockchainer. 11:06:02.887 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@7d1a9568: T(3b6c, 5500/2, 2021-05-11 18:06:02) is published. 11:06:02.892 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(3b6c, 5500/2, 2021-05-11 18:06:02) to transaction queue. 11:06:03.290 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(36fa, 5500/3, 2021-05-11 18:06:03) to transaction queue. 11:06:04.097 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 22000 millis 11:06:04.097 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:06:04.098 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(ac20, 2500/1, 2021-05-11 18:05:02), T(7634, 5000/3, 2021-05-11 18:05:03), T(a545, 2500/1, 2021-05-11 18:05:17), T(6e8e, 6500/3, 2021-05-11 18:05:18), T(862f, 1000/1, 2021-05-11 18:05:32), T(a81d, 7000/3, 2021-05-11 18:05:33), T(69d4, 4500/2, 2021-05-11 18:05:47), T(0060, 2000/1, 2021-05-11 18:05:47), T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03)) 11:06:04.099 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:06:12.466 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@7d1a9568: BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119) is valid. Updating blockchain. 11:06:12.466 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:17.029 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@7d1a9568: BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357) is valid. Updating blockchain. 11:06:17.029 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:17.372 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(4919, 2500/2, 2021-05-11 18:06:17) to transaction queue. 11:06:17.893 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(a892, 4500/2, 2021-05-11 18:06:17) to Blockchainer. 11:06:17.894 [blockchain-akka.actor.default-dispatcher-28] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@7d1a9568: T(a892, 4500/2, 2021-05-11 18:06:17) is published. 11:06:17.895 [blockchain-akka.actor.default-dispatcher-28] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(a892, 4500/2, 2021-05-11 18:06:17) to transaction queue. 11:06:18.291 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(45db, 2500/2, 2021-05-11 18:06:18) to transaction queue. 11:06:24.093 [blockchain-akka.actor.default-dispatcher-5] ERROR akkablockchain.actor.Blockchainer - [Req.Mining] akkablockchain.actor.Blockchainer@7d1a9568: ERROR: java.util.concurrent.TimeoutException: Ask timed out on [Actor[akka://blockchain/user/blockchainer/miner#-450328640]] after [20000 ms]. Message of type [akkablockchain.actor.Miner$Mine]. A typical reason for `AskTimeoutException` is that the recipient actor didn't send a reply. 11:06:24.094 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:06:26.153 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 14000 millis 11:06:26.153 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:06:26.154 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(7634, 5000/3, 2021-05-11 18:05:03), T(a545, 2500/1, 2021-05-11 18:05:17), T(6e8e, 6500/3, 2021-05-11 18:05:18), T(862f, 1000/1, 2021-05-11 18:05:32), T(a81d, 7000/3, 2021-05-11 18:05:33), T(69d4, 4500/2, 2021-05-11 18:05:47), T(0060, 2000/1, 2021-05-11 18:05:47), T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03), T(4919, 2500/2, 2021-05-11 18:06:17), T(a892, 4500/2, 2021-05-11 18:06:17), T(45db, 2500/2, 2021-05-11 18:06:18)) 11:06:26.155 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357), BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:06:32.378 [blockchain-akka.actor.default-dispatcher-28] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(850c, 5000/3, 2021-05-11 18:06:32) to transaction queue. 11:06:32.899 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(bd5f, 4000/2, 2021-05-11 18:06:32) to Blockchainer. 11:06:32.900 [blockchain-akka.actor.default-dispatcher-28] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@7d1a9568: T(bd5f, 4000/2, 2021-05-11 18:06:32) is published. 11:06:32.900 [blockchain-akka.actor.default-dispatcher-28] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(bd5f, 4000/2, 2021-05-11 18:06:32) to transaction queue. 11:06:33.295 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@7d1a9568: Appended T(72cc, 4500/3, 2021-05-11 18:06:33) to transaction queue. 11:06:35.314 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@7d1a9568: BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378) is valid. Updating blockchain. 11:06:35.314 [blockchain-akka.actor.default-dispatcher-28] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:37.938 [blockchain-akka.actor.default-dispatcher-28] WARN akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@7d1a9568: BLK(7V0m, T(69d4, 4599/3), 2021-05-11 18:06:32, 3, 7774969) isn’t longer than existing blockchain! Blockchain not updated. 11:06:37.938 [blockchain-akka.actor.default-dispatcher-28] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:40.177 [blockchain-akka.actor.default-dispatcher-5] ERROR akkablockchain.actor.Miner - [Mining] Miner.Mine(BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378), T(7634, 5000/3, 2021-05-11 18:05:03)) received but akkablockchain.actor.Miner@596f15b4 is busy! 11:06:40.208 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 21000 millis 11:06:40.208 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:06:40.209 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(a545, 2500/1, 2021-05-11 18:05:17), T(6e8e, 6500/3, 2021-05-11 18:05:18), T(862f, 1000/1, 2021-05-11 18:05:32), T(a81d, 7000/3, 2021-05-11 18:05:33), T(69d4, 4500/2, 2021-05-11 18:05:47), T(0060, 2000/1, 2021-05-11 18:05:47), T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03), T(4919, 2500/2, 2021-05-11 18:06:17), T(a892, 4500/2, 2021-05-11 18:06:17), T(45db, 2500/2, 2021-05-11 18:06:18), T(850c, 5000/3, 2021-05-11 18:06:32), T(bd5f, 4000/2, 2021-05-11 18:06:32), T(72cc, 4500/3, 2021-05-11 18:06:33)) 11:06:40.209 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378), BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357), BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:06:43.637 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:06:43.638 [blockchain-akka.actor.default-dispatcher-5] ERROR akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@7d1a9568: ERROR: BLK(jy21, T(ac20, 2599/2), 2021-05-11 18:06:26, 3, 24589944) is invalid! 11:06:43.638 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. ^C <<< <<<--- User terminated program on the node (seed node #1) <<< [warn] Canceling execution... Cancelled: runMain akkablockchain.Main 2551 src/main/resources/keys/account0_public.pem [error] Cancelled: runMain akkablockchain.Main 2551 src/main/resources/keys/account0_public.pem [error] Use 'last' for the full log. 11:06:46.552 [blockchain-akka.actor.default-dispatcher-18] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Marked address [akka://blockchain@127.0.0.1:2551] as [Leaving] 11:06:46.770 [blockchain-akka.actor.default-dispatcher-18] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Leader is moving node [akka://blockchain@127.0.0.1:2551] to [Exiting] 11:06:46.772 [blockchain-akka.actor.default-dispatcher-18] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Exiting completed 11:06:46.777 [blockchain-akka.actor.default-dispatcher-18] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Shutting down... 11:06:46.780 [blockchain-akka.actor.default-dispatcher-18] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2551] - Successfully shut down 11:06:46.787 [blockchain-akka.actor.internal-dispatcher-12] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2551] - terminated/removed 11:06:46.791 [blockchain-akka.actor.internal-dispatcher-12] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - Cancel all timers 11:06:46.795 [blockchain-akka.actor.default-dispatcher-17] INFO akka.actor.LocalActorRef - Message [akka.cluster.GossipEnvelope] from Actor[akka://blockchain@127.0.0.1:2552/system/cluster/core/daemon#1959897872] to Actor[akka://blockchain/system/cluster/core/daemon#-236218873] was not delivered. [1] dead letters encountered. If this is not an expected behavior then Actor[akka://blockchain/system/cluster/core/daemon#-236218873] may have terminated unexpectedly. This logging can be turned off or adjusted with configuration settings 'akka.log-dead-letters' and 'akka.log-dead-letters-during-shutdown'. 11:06:46.799 [blockchain-akka.actor.default-dispatcher-31] INFO akka.remote.RemoteActorRefProvider$RemotingTerminator - Shutting down remote daemon. 11:06:46.801 [blockchain-akka.actor.default-dispatcher-31] INFO akka.remote.RemoteActorRefProvider$RemotingTerminator - Remote daemon shut down; proceeding with flushing remote transports. 11:06:46.841 [blockchain-akka.actor.default-dispatcher-31] WARN akka.stream.Materializer - [outbound connection to [akka://blockchain@127.0.0.1:2553], message stream] Upstream failed, cause: StreamTcpException: The connection has been aborted 11:06:46.841 [blockchain-akka.actor.default-dispatcher-31] WARN akka.stream.Materializer - [outbound connection to [akka://blockchain@127.0.0.1:2552], control stream] Upstream failed, cause: StreamTcpException: The connection has been aborted 11:06:46.845 [blockchain-akka.actor.default-dispatcher-31] WARN akka.stream.Materializer - [outbound connection to [akka://blockchain@127.0.0.1:2553], control stream] Upstream failed, cause: StreamTcpException: The connection has been aborted 11:06:46.846 [blockchain-akka.actor.default-dispatcher-31] WARN akka.stream.Materializer - [outbound connection to [akka://blockchain@127.0.0.1:2552], message stream] Upstream failed, cause: StreamTcpException: The connection has been aborted 11:06:46.858 [blockchain-akka.actor.default-dispatcher-31] INFO akka.remote.RemoteActorRefProvider$RemotingTerminator - Remoting shut down. |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 |
### Console Log: CLUSTER NODE #2 $ sbt "runMain akkablockchain.Main 2552 src/main/resources/keys/account1_public.pem" [info] running akkablockchain.Main 2552 src/main/resources/keys/account1_public.pem 11:04:59.389 [blockchain-akka.actor.default-dispatcher-3] INFO akka.event.slf4j.Slf4jLogger - Slf4jLogger started SLF4J: A number (1) of logging calls during the initialization phase have been intercepted and are SLF4J: now being replayed. These are subject to the filtering rules of the underlying logging system. SLF4J: See also http://www.slf4j.org/codes.html#replay 11:04:59.735 [blockchain-akka.actor.default-dispatcher-3] INFO akka.remote.artery.tcp.ArteryTcpTransport - Remoting started with transport [Artery tcp]; listening on address [akka://blockchain@127.0.0.1:2552] with UID [-3750460307660254480] 11:04:59.762 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Starting up, Akka version [2.6.13] ... 11:04:59.892 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Registered cluster JMX MBean [akka:type=Cluster] 11:04:59.892 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Started up successfully <<< <<<--- Seed node #2, bound to port# 2552, joined the cluster <<< 11:04:59.918 [blockchain-akka.actor.default-dispatcher-5] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - No downing-provider-class configured, manual cluster downing required, see https://doc.akka.io/docs/akka/current/typed/cluster.html#downing 11:05:00.243 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] idle: Got akka.actor.LightArrayRevolverScheduler$TaskHolder@637a1375 11:05:00.248 [blockchain-akka.actor.default-dispatcher-5] DEBUG akka.actor.typed.pubsub.Topic$ - Starting up pub-sub topic [new-transactions] for messages of type [akkablockchain.actor.Blockchainer$Req] 11:05:00.248 [blockchain-akka.actor.default-dispatcher-17] DEBUG akka.actor.typed.pubsub.Topic$ - Starting up pub-sub topic [new-block] for messages of type [akkablockchain.actor.Blockchainer$Req] 11:05:00.257 [blockchain-akka.actor.default-dispatcher-17] DEBUG akka.actor.typed.pubsub.Topic$ - Local subscriber [Actor[akka://blockchain/user/blockchainer#-359491379]] added, went from no subscribers to one, subscribing to receptionist 11:05:00.257 [blockchain-akka.actor.default-dispatcher-5] DEBUG akka.actor.typed.pubsub.Topic$ - Local subscriber [Actor[akka://blockchain/user/blockchainer#-359491379]] added, went from no subscribers to one, subscribing to receptionist 11:05:00.276 [blockchain-akka.actor.internal-dispatcher-12] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Actor was registered: [ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block)] [akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480] 11:05:00.279 [blockchain-akka.actor.default-dispatcher-5] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set()] 11:05:00.279 [blockchain-akka.actor.default-dispatcher-15] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set()] 11:05:00.280 [blockchain-akka.actor.internal-dispatcher-12] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Actor was registered: [ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions)] [akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480] 11:05:00.541 [blockchain-akka.actor.default-dispatcher-15] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Received InitJoinAck message from [Actor[akka://blockchain@127.0.0.1:2551/system/cluster/core/daemon#-236218873]] to [akka://blockchain@127.0.0.1:2552] 11:05:00.625 [blockchain-akka.actor.default-dispatcher-15] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Welcome from [akka://blockchain@127.0.0.1:2551] 11:05:00.637 [blockchain-akka.actor.internal-dispatcher-12] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Node added [UniqueAddress(akka://blockchain@127.0.0.1:2551,5401420401358518049)] 11:05:00.786 [blockchain-akka.actor.internal-dispatcher-14] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Change from replicator: [Map(ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block) -> Set(akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480), ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions) -> Set(akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480))], changes: [(new-block,[akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480]), (new-transactions,[akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480])], tombstones [] 11:05:00.787 [blockchain-akka.actor.default-dispatcher-15] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180])] 11:05:00.788 [blockchain-akka.actor.default-dispatcher-5] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065])] 11:05:02.342 [blockchain-akka.actor.internal-dispatcher-14] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Change from replicator: [Map(ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block) -> Set(akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049), ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions) -> Set(akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049))], changes: [(new-block,[akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049]), (new-transactions,[akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049])], tombstones [] 11:05:02.343 [blockchain-akka.actor.default-dispatcher-5] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180], Actor[akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewBlock#-497024677])] 11:05:02.343 [blockchain-akka.actor.default-dispatcher-15] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065], Actor[akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewTransactions#-2092003166])] 11:05:02.913 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(ac20, 2500/1, 2021-05-11 18:05:02) to transaction queue. 11:05:03.270 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(7634, 5000/3, 2021-05-11 18:05:03) to Blockchainer. 11:05:03.272 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(7634, 5000/3, 2021-05-11 18:05:03) is published. 11:05:03.273 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(7634, 5000/3, 2021-05-11 18:05:03) to transaction queue. 11:05:15.772 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128) is valid. Updating blockchain. 11:05:15.773 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:05:17.875 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(a545, 2500/1, 2021-05-11 18:05:17) to transaction queue. 11:05:18.260 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(6e8e, 6500/3, 2021-05-11 18:05:18) to Blockchainer. 11:05:18.261 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(6e8e, 6500/3, 2021-05-11 18:05:18) is published. 11:05:18.262 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(6e8e, 6500/3, 2021-05-11 18:05:18) to transaction queue. 11:05:30.283 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 15000 millis 11:05:30.284 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:05:30.285 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(ac20, 2500/1, 2021-05-11 18:05:02), T(7634, 5000/3, 2021-05-11 18:05:03), T(a545, 2500/1, 2021-05-11 18:05:17), T(6e8e, 6500/3, 2021-05-11 18:05:18)) 11:05:30.286 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:05:32.882 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(862f, 1000/1, 2021-05-11 18:05:32) to transaction queue. 11:05:33.276 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(a81d, 7000/3, 2021-05-11 18:05:33) to Blockchainer. 11:05:33.279 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(a81d, 7000/3, 2021-05-11 18:05:33) is published. 11:05:33.281 [blockchain-akka.actor.default-dispatcher-5] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(a81d, 7000/3, 2021-05-11 18:05:33) to transaction queue. 11:05:41.922 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242) is valid. Updating blockchain. 11:05:41.922 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:05:44.516 [blockchain-akka.actor.default-dispatcher-16] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Received InitJoin message from [Actor[akka://blockchain@127.0.0.1:2553/system/cluster/core/daemon/joinSeedNodeProcess-1#-236485565]] to [akka://blockchain@127.0.0.1:2552] 11:05:44.516 [blockchain-akka.actor.default-dispatcher-16] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Sending InitJoinAck message from node [akka://blockchain@127.0.0.1:2552] to [Actor[akka://blockchain@127.0.0.1:2553/system/cluster/core/daemon/joinSeedNodeProcess-1#-236485565]] (version [2.6.13]) 11:05:44.760 [blockchain-akka.actor.internal-dispatcher-4] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Node added [UniqueAddress(akka://blockchain@127.0.0.1:2553,5558056305511553349)] 11:05:44.997 [blockchain-akka.actor.internal-dispatcher-26] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Change from replicator: [Map(ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block) -> Set(akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049, akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349), ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions) -> Set(akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049, akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349))], changes: [(new-block,[akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049, akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349]), (new-transactions,[akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049, akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349])], tombstones [] 11:05:44.998 [blockchain-akka.actor.default-dispatcher-16] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180], Actor[akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewBlock#-497024677], Actor[akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewBlock#1714452195])] 11:05:44.998 [blockchain-akka.actor.default-dispatcher-15] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065], Actor[akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewTransactions#-2092003166], Actor[akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewTransactions#-2035531976])] 11:05:45.335 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 14000 millis 11:05:45.335 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:05:45.336 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(7634, 5000/3, 2021-05-11 18:05:03), T(a545, 2500/1, 2021-05-11 18:05:17), T(6e8e, 6500/3, 2021-05-11 18:05:18), T(862f, 1000/1, 2021-05-11 18:05:32), T(a81d, 7000/3, 2021-05-11 18:05:33)) 11:05:45.337 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:05:47.394 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(69d4, 4500/2, 2021-05-11 18:05:47) to transaction queue. 11:05:47.886 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(0060, 2000/1, 2021-05-11 18:05:47) to transaction queue. 11:05:48.280 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(bca5, 4000/3, 2021-05-11 18:05:48) to Blockchainer. 11:05:48.280 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(bca5, 4000/3, 2021-05-11 18:05:48) is published. 11:05:48.281 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(bca5, 4000/3, 2021-05-11 18:05:48) to transaction queue. 11:05:53.520 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:05:53.522 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567) is valid. Updating blockchain. 11:05:53.522 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:05:59.390 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 15000 millis 11:05:59.391 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:05:59.392 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(a545, 2500/1, 2021-05-11 18:05:17), T(6e8e, 6500/3, 2021-05-11 18:05:18), T(862f, 1000/1, 2021-05-11 18:05:32), T(a81d, 7000/3, 2021-05-11 18:05:33), T(69d4, 4500/2, 2021-05-11 18:05:47), T(0060, 2000/1, 2021-05-11 18:05:47), T(bca5, 4000/3, 2021-05-11 18:05:48)) 11:05:59.392 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:06:02.372 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(b137, 3500/2, 2021-05-11 18:06:02) to transaction queue. 11:06:02.891 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(3b6c, 5500/2, 2021-05-11 18:06:02) to transaction queue. 11:06:03.284 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(36fa, 5500/3, 2021-05-11 18:06:03) to Blockchainer. 11:06:03.285 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(36fa, 5500/3, 2021-05-11 18:06:03) is published. 11:06:03.286 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(36fa, 5500/3, 2021-05-11 18:06:03) to transaction queue. 11:06:12.455 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:06:12.457 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119) is valid. Updating blockchain. 11:06:12.458 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:14.443 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 17000 millis 11:06:14.444 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:06:14.445 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(6e8e, 6500/3, 2021-05-11 18:05:18), T(862f, 1000/1, 2021-05-11 18:05:32), T(a81d, 7000/3, 2021-05-11 18:05:33), T(69d4, 4500/2, 2021-05-11 18:05:47), T(0060, 2000/1, 2021-05-11 18:05:47), T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03)) 11:06:14.446 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:06:17.019 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:06:17.021 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357) is valid. Updating blockchain. 11:06:17.022 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:17.372 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(4919, 2500/2, 2021-05-11 18:06:17) to transaction queue. 11:06:17.896 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(a892, 4500/2, 2021-05-11 18:06:17) to transaction queue. 11:06:18.288 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(45db, 2500/2, 2021-05-11 18:06:18) to Blockchainer. 11:06:18.289 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(45db, 2500/2, 2021-05-11 18:06:18) is published. 11:06:18.289 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(45db, 2500/2, 2021-05-11 18:06:18) to transaction queue. 11:06:31.500 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 17000 millis 11:06:31.500 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:06:31.501 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(862f, 1000/1, 2021-05-11 18:05:32), T(a81d, 7000/3, 2021-05-11 18:05:33), T(69d4, 4500/2, 2021-05-11 18:05:47), T(0060, 2000/1, 2021-05-11 18:05:47), T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03), T(4919, 2500/2, 2021-05-11 18:06:17), T(a892, 4500/2, 2021-05-11 18:06:17), T(45db, 2500/2, 2021-05-11 18:06:18)) 11:06:31.503 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357), BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:06:32.378 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(850c, 5000/3, 2021-05-11 18:06:32) to transaction queue. 11:06:32.902 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(bd5f, 4000/2, 2021-05-11 18:06:32) to transaction queue. 11:06:33.292 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(72cc, 4500/3, 2021-05-11 18:06:33) to Blockchainer. 11:06:33.292 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(72cc, 4500/3, 2021-05-11 18:06:33) is published. 11:06:33.293 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(72cc, 4500/3, 2021-05-11 18:06:33) to transaction queue. 11:06:35.305 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:06:35.307 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378) is valid. Updating blockchain. 11:06:35.307 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:37.940 [blockchain-akka.actor.default-dispatcher-17] WARN akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: BLK(7V0m, T(69d4, 4599/3), 2021-05-11 18:06:32, 3, 7774969) isn’t longer than existing blockchain! Blockchain not updated. 11:06:37.941 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:43.645 [blockchain-akka.actor.default-dispatcher-17] ERROR akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: ERROR: BLK(jy21, T(ac20, 2599/2), 2021-05-11 18:06:26, 3, 24589944) is invalid! 11:06:43.645 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:46.783 [blockchain-akka.actor.default-dispatcher-16] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Exiting confirmed [akka://blockchain@127.0.0.1:2551] 11:06:46.986 [blockchain-akka.actor.default-dispatcher-16] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - is the new leader among reachable nodes (more leaders may exist) <<< <<<-- Marked seed note #1 as `exiting confirmed` and made itself (seed node #2) the new cluster `leader` <<< 11:06:47.379 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(0e7e, 1000/1, 2021-05-11 18:06:47) to transaction queue. 11:06:47.827 [blockchain-akka.actor.default-dispatcher-17] INFO akka.remote.artery.Association - Association to [akka://blockchain@127.0.0.1:2551] having UID [5401420401358518049] has been stopped. All messages to this UID will be delivered to dead letters. Reason: ActorSystem terminated 11:06:48.017 [blockchain-akka.actor.default-dispatcher-17] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Leader is removing confirmed Exiting node [akka://blockchain@127.0.0.1:2551] 11:06:48.021 [blockchain-akka.actor.internal-dispatcher-25] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Node with registered services removed [UniqueAddress(akka://blockchain@127.0.0.1:2551,5401420401358518049)] 11:06:48.021 [blockchain-akka.actor.internal-dispatcher-25] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Leader node observed removed node [UniqueAddress(akka://blockchain@127.0.0.1:2551,5401420401358518049)] 11:06:48.021 [blockchain-akka.actor.default-dispatcher-17] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180], Actor[akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewBlock#1714452195])] 11:06:48.021 [blockchain-akka.actor.default-dispatcher-16] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065], Actor[akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewTransactions#-2035531976])] 11:06:48.026 [blockchain-akka.actor.internal-dispatcher-25] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Node(s) removed [UniqueAddress(akka://blockchain@127.0.0.1:2551,5401420401358518049)], updating registry removing entries: [new-block -> [akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049],new-transactions -> [akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049]] 11:06:48.295 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(3bfc, 2000/1, 2021-05-11 18:06:48) to Blockchainer. 11:06:48.296 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(3bfc, 2000/1, 2021-05-11 18:06:48) is published. 11:06:48.296 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(3bfc, 2000/1, 2021-05-11 18:06:48) to transaction queue. 11:06:48.456 [blockchain-akka.actor.internal-dispatcher-12] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Change from replicator: [Map(ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block) -> Set(akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349, akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480), ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions) -> Set(akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349, akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480))], changes: [(new-block,[akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349, akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480]), (new-transactions,[akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349, akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480])], tombstones [] 11:06:48.457 [blockchain-akka.actor.default-dispatcher-17] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewBlock#1714452195], Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180])] 11:06:48.457 [blockchain-akka.actor.default-dispatcher-16] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewTransactions#-2035531976], Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065])] 11:06:48.556 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 21000 millis 11:06:48.557 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:06:48.557 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(a81d, 7000/3, 2021-05-11 18:05:33), T(69d4, 4500/2, 2021-05-11 18:05:47), T(0060, 2000/1, 2021-05-11 18:05:47), T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03), T(4919, 2500/2, 2021-05-11 18:06:17), T(a892, 4500/2, 2021-05-11 18:06:17), T(45db, 2500/2, 2021-05-11 18:06:18), T(850c, 5000/3, 2021-05-11 18:06:32), T(bd5f, 4000/2, 2021-05-11 18:06:32), T(72cc, 4500/3, 2021-05-11 18:06:33), T(0e7e, 1000/1, 2021-05-11 18:06:47), T(3bfc, 2000/1, 2021-05-11 18:06:48)) 11:06:48.558 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378), BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357), BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:06:59.444 [blockchain-akka.actor.default-dispatcher-29] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: BLK(AsCZ, T(0060, 2099/2), 2021-05-11 18:06:51, 3, 12440412) is valid. Updating blockchain. 11:06:59.444 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:07:02.385 [blockchain-akka.actor.default-dispatcher-29] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(130f, 4000/2, 2021-05-11 18:07:02) to transaction queue. 11:07:03.301 [blockchain-akka.actor.default-dispatcher-29] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(6179, 4000/2, 2021-05-11 18:07:03) to Blockchainer. 11:07:03.302 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(6179, 4000/2, 2021-05-11 18:07:03) is published. 11:07:03.303 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(6179, 4000/2, 2021-05-11 18:07:03) to transaction queue. 11:07:08.552 [blockchain-akka.actor.default-dispatcher-29] ERROR akkablockchain.actor.Blockchainer - [Req.Mining] akkablockchain.actor.Blockchainer@650585f8: ERROR: java.util.concurrent.TimeoutException: Ask timed out on [Actor[akka://blockchain/user/blockchainer/miner#395585846]] after [20000 ms]. Message of type [akkablockchain.actor.Miner$Mine]. A typical reason for `AskTimeoutException` is that the recipient actor didn't send a reply. 11:07:08.552 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:07:09.612 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 20000 millis 11:07:09.613 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:07:09.614 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(69d4, 4500/2, 2021-05-11 18:05:47), T(0060, 2000/1, 2021-05-11 18:05:47), T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03), T(4919, 2500/2, 2021-05-11 18:06:17), T(a892, 4500/2, 2021-05-11 18:06:17), T(45db, 2500/2, 2021-05-11 18:06:18), T(850c, 5000/3, 2021-05-11 18:06:32), T(bd5f, 4000/2, 2021-05-11 18:06:32), T(72cc, 4500/3, 2021-05-11 18:06:33), T(0e7e, 1000/1, 2021-05-11 18:06:47), T(3bfc, 2000/1, 2021-05-11 18:06:48), T(130f, 4000/2, 2021-05-11 18:07:02), T(6179, 4000/2, 2021-05-11 18:07:03)) 11:07:09.614 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(AsCZ, T(0060, 2099/2), 2021-05-11 18:06:51, 3, 12440412), BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378), BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357), BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:07:11.411 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: BLK(G7b0, T(bca5, 4099/4), 2021-05-11 18:07:06, 3, 7482632) is valid. Updating blockchain. 11:07:11.411 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:07:11.433 [blockchain-akka.actor.default-dispatcher-29] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:07:11.435 [blockchain-akka.actor.default-dispatcher-29] WARN akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: BLK(WzL+, T(a81d, 7099/4), 2021-05-11 18:07:09, 3, 2734351) isn’t longer than existing blockchain! Blockchain not updated. 11:07:11.436 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:07:17.391 [blockchain-akka.actor.default-dispatcher-29] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(3ecc, 3000/2, 2021-05-11 18:07:17) to transaction queue. 11:07:18.306 [blockchain-akka.actor.default-dispatcher-29] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(6d51, 3000/1, 2021-05-11 18:07:18) to Blockchainer. 11:07:18.306 [blockchain-akka.actor.default-dispatcher-29] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(6d51, 3000/1, 2021-05-11 18:07:18) is published. 11:07:18.307 [blockchain-akka.actor.default-dispatcher-29] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(6d51, 3000/1, 2021-05-11 18:07:18) to transaction queue. 11:07:29.670 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 24000 millis 11:07:29.670 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:07:29.671 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(0060, 2000/1, 2021-05-11 18:05:47), T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03), T(4919, 2500/2, 2021-05-11 18:06:17), T(a892, 4500/2, 2021-05-11 18:06:17), T(45db, 2500/2, 2021-05-11 18:06:18), T(850c, 5000/3, 2021-05-11 18:06:32), T(bd5f, 4000/2, 2021-05-11 18:06:32), T(72cc, 4500/3, 2021-05-11 18:06:33), T(0e7e, 1000/1, 2021-05-11 18:06:47), T(3bfc, 2000/1, 2021-05-11 18:06:48), T(130f, 4000/2, 2021-05-11 18:07:02), T(6179, 4000/2, 2021-05-11 18:07:03), T(3ecc, 3000/2, 2021-05-11 18:07:17), T(6d51, 3000/1, 2021-05-11 18:07:18)) 11:07:29.672 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(G7b0, T(bca5, 4099/4), 2021-05-11 18:07:06, 3, 7482632), BLK(AsCZ, T(0060, 2099/2), 2021-05-11 18:06:51, 3, 12440412), BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378), BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357), BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:07:32.394 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(4684, 5000/2, 2021-05-11 18:07:32) to transaction queue. 11:07:33.309 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(f1fe, 1500/1, 2021-05-11 18:07:33) to Blockchainer. 11:07:33.310 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(f1fe, 1500/1, 2021-05-11 18:07:33) is published. 11:07:33.311 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(f1fe, 1500/1, 2021-05-11 18:07:33) to transaction queue. 11:07:33.911 [blockchain-akka.actor.default-dispatcher-31] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Leader is moving node [akka://blockchain@127.0.0.1:2553] to [Exiting] <<< <<<--- Marked node #3 as `exiting` <<< 11:07:33.928 [blockchain-akka.actor.default-dispatcher-31] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Exiting confirmed [akka://blockchain@127.0.0.1:2553] 11:07:34.930 [blockchain-akka.actor.default-dispatcher-31] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Leader is removing confirmed Exiting node [akka://blockchain@127.0.0.1:2553] 11:07:34.931 [blockchain-akka.actor.internal-dispatcher-7] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Node with registered services removed [UniqueAddress(akka://blockchain@127.0.0.1:2553,5558056305511553349)] 11:07:34.931 [blockchain-akka.actor.internal-dispatcher-7] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Leader node observed removed node [UniqueAddress(akka://blockchain@127.0.0.1:2553,5558056305511553349)] 11:07:34.931 [blockchain-akka.actor.default-dispatcher-31] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065])] 11:07:34.931 [blockchain-akka.actor.default-dispatcher-3] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180])] 11:07:34.932 [blockchain-akka.actor.internal-dispatcher-7] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Node(s) removed [UniqueAddress(akka://blockchain@127.0.0.1:2553,5558056305511553349)], updating registry removing entries: [new-block -> [akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349],new-transactions -> [akka://blockchain@127.0.0.1:2553/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349]] 11:07:34.961 [blockchain-akka.actor.default-dispatcher-3] INFO akka.remote.artery.Association - Association to [akka://blockchain@127.0.0.1:2553] having UID [5558056305511553349] has been stopped. All messages to this UID will be delivered to dead letters. Reason: ActorSystem terminated 11:07:35.270 [blockchain-akka.actor.internal-dispatcher-13] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - Change from replicator: [Map(ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block) -> Set(akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480), ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions) -> Set(akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480))], changes: [(new-block,[akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480]), (new-transactions,[akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480])], tombstones [] 11:07:35.271 [blockchain-akka.actor.default-dispatcher-31] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-1565562065])] 11:07:35.271 [blockchain-akka.actor.default-dispatcher-3] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#1064341180])] 11:07:46.075 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:07:46.077 [blockchain-akka.actor.default-dispatcher-31] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@650585f8: BLK(LQ6u, T(69d4, 4599/3), 2021-05-11 18:07:29, 3, 24417860) is valid. Updating blockchain. 11:07:46.077 [blockchain-akka.actor.default-dispatcher-31] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:07:48.317 [blockchain-akka.actor.default-dispatcher-31] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(1dc1, 7500/3, 2021-05-11 18:07:48) to Blockchainer. 11:07:48.318 [blockchain-akka.actor.default-dispatcher-31] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(1dc1, 7500/3, 2021-05-11 18:07:48) is published. 11:07:48.318 [blockchain-akka.actor.default-dispatcher-31] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(1dc1, 7500/3, 2021-05-11 18:07:48) to transaction queue. 11:07:53.726 [blockchain-akka.actor.default-dispatcher-31] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 21000 millis 11:07:53.726 [blockchain-akka.actor.default-dispatcher-31] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:07:53.727 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03), T(4919, 2500/2, 2021-05-11 18:06:17), T(a892, 4500/2, 2021-05-11 18:06:17), T(45db, 2500/2, 2021-05-11 18:06:18), T(850c, 5000/3, 2021-05-11 18:06:32), T(bd5f, 4000/2, 2021-05-11 18:06:32), T(72cc, 4500/3, 2021-05-11 18:06:33), T(0e7e, 1000/1, 2021-05-11 18:06:47), T(3bfc, 2000/1, 2021-05-11 18:06:48), T(130f, 4000/2, 2021-05-11 18:07:02), T(6179, 4000/2, 2021-05-11 18:07:03), T(3ecc, 3000/2, 2021-05-11 18:07:17), T(6d51, 3000/1, 2021-05-11 18:07:18), T(4684, 5000/2, 2021-05-11 18:07:32), T(f1fe, 1500/1, 2021-05-11 18:07:33), T(1dc1, 7500/3, 2021-05-11 18:07:48)) 11:07:53.727 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(LQ6u, T(69d4, 4599/3), 2021-05-11 18:07:29, 3, 24417860), BLK(G7b0, T(bca5, 4099/4), 2021-05-11 18:07:06, 3, 7482632), BLK(AsCZ, T(0060, 2099/2), 2021-05-11 18:06:51, 3, 12440412), BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378), BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357), BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:08:03.321 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(d4e2, 5000/3, 2021-05-11 18:08:03) to Blockchainer. 11:08:03.322 [blockchain-akka.actor.default-dispatcher-31] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@650585f8: T(d4e2, 5000/3, 2021-05-11 18:08:03) is published. 11:08:03.322 [blockchain-akka.actor.default-dispatcher-31] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@650585f8: Appended T(d4e2, 5000/3, 2021-05-11 18:08:03) to transaction queue. ^C <<< <<<--- User terminated program on the node (seed node #2) <<< [warn] Canceling execution... Cancelled: runMain akkablockchain.Main 2552 src/main/resources/keys/account1_public.pem [error] Cancelled: runMain akkablockchain.Main 2552 src/main/resources/keys/account1_public.pem [error] Use 'last' for the full log. 11:08:04.715 [blockchain-akka.actor.default-dispatcher-31] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Marked address [akka://blockchain@127.0.0.1:2552] as [Leaving] 11:08:05.535 [blockchain-akka.actor.default-dispatcher-31] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Leader is moving node [akka://blockchain@127.0.0.1:2552] to [Exiting] 11:08:05.535 [blockchain-akka.actor.default-dispatcher-31] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Exiting completed 11:08:05.537 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Shutting down... 11:08:05.539 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2552] - Successfully shut down 11:08:05.542 [blockchain-akka.actor.internal-dispatcher-13] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2552] - terminated/removed 11:08:05.546 [blockchain-akka.actor.internal-dispatcher-13] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - Cancel all timers 11:08:05.556 [blockchain-akka.actor.default-dispatcher-3] INFO akka.remote.RemoteActorRefProvider$RemotingTerminator - Shutting down remote daemon. 11:08:05.558 [blockchain-akka.actor.default-dispatcher-3] INFO akka.remote.RemoteActorRefProvider$RemotingTerminator - Remote daemon shut down; proceeding with flushing remote transports. 11:08:05.577 [blockchain-akka.actor.default-dispatcher-3] INFO akka.remote.RemoteActorRefProvider$RemotingTerminator - Remoting shut down. |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 |
### Console Log: CLUSTER NODE #3 $ sbt "runMain akkablockchain.Main 2553 src/main/resources/keys/account2_public.pem" [info] running akkablockchain.Main 2553 src/main/resources/keys/account2_public.pem 11:05:43.560 [blockchain-akka.actor.default-dispatcher-3] INFO akka.event.slf4j.Slf4jLogger - Slf4jLogger started SLF4J: A number (1) of logging calls during the initialization phase have been intercepted and are SLF4J: now being replayed. These are subject to the filtering rules of the underlying logging system. SLF4J: See also http://www.slf4j.org/codes.html#replay 11:05:43.880 [blockchain-akka.actor.default-dispatcher-3] INFO akka.remote.artery.tcp.ArteryTcpTransport - Remoting started with transport [Artery tcp]; listening on address [akka://blockchain@127.0.0.1:2553] with UID [5558056305511553349] 11:05:43.903 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2553] - Starting up, Akka version [2.6.13] ... 11:05:44.013 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2553] - Registered cluster JMX MBean [akka:type=Cluster] 11:05:44.013 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2553] - Started up successfully <<< <<<--- Node #3, bound to port# 2553, joined the cluster <<< 11:05:44.037 [blockchain-akka.actor.default-dispatcher-3] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2553] - No downing-provider-class configured, manual cluster downing required, see https://doc.akka.io/docs/akka/current/typed/cluster.html#downing 11:05:44.345 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] idle: Got akka.actor.LightArrayRevolverScheduler$TaskHolder@5bbde918 11:05:44.349 [blockchain-akka.actor.default-dispatcher-3] DEBUG akka.actor.typed.pubsub.Topic$ - Starting up pub-sub topic [new-transactions] for messages of type [akkablockchain.actor.Blockchainer$Req] 11:05:44.349 [blockchain-akka.actor.default-dispatcher-17] DEBUG akka.actor.typed.pubsub.Topic$ - Starting up pub-sub topic [new-block] for messages of type [akkablockchain.actor.Blockchainer$Req] 11:05:44.368 [blockchain-akka.actor.default-dispatcher-17] DEBUG akka.actor.typed.pubsub.Topic$ - Local subscriber [Actor[akka://blockchain/user/blockchainer#-13396684]] added, went from no subscribers to one, subscribing to receptionist 11:05:44.368 [blockchain-akka.actor.default-dispatcher-3] DEBUG akka.actor.typed.pubsub.Topic$ - Local subscriber [Actor[akka://blockchain/user/blockchainer#-13396684]] added, went from no subscribers to one, subscribing to receptionis 11:05:44.386 [blockchain-akka.actor.internal-dispatcher-4] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2553] - Actor was registered: [ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions)] [akka://blockchain/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349] 11:05:44.388 [blockchain-akka.actor.default-dispatcher-3] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set()] 11:05:44.388 [blockchain-akka.actor.default-dispatcher-15] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set()] 11:05:44.390 [blockchain-akka.actor.internal-dispatcher-4] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2553] - Actor was registered: [ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block)] [akka://blockchain/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349] 11:05:44.575 [blockchain-akka.actor.default-dispatcher-15] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2553] - Received InitJoinAck message from [Actor[akka://blockchain@127.0.0.1:2551/system/cluster/core/daemon#-236218873]] to [akka://blockchain@127.0.0.1:2553] 11:05:44.628 [blockchain-akka.actor.default-dispatcher-15] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2553] - Welcome from [akka://blockchain@127.0.0.1:2551] 11:05:44.642 [blockchain-akka.actor.internal-dispatcher-12] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2553] - Node added [UniqueAddress(akka://blockchain@127.0.0.1:2551,5401420401358518049)] 11:05:44.644 [blockchain-akka.actor.internal-dispatcher-12] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2553] - Node added [UniqueAddress(akka://blockchain@127.0.0.1:2552,-3750460307660254480)] 11:05:44.901 [blockchain-akka.actor.internal-dispatcher-5] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2553] - Change from replicator: [Map(ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions) -> Set(akka://blockchain/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349), ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block) -> Set(akka://blockchain/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349))], changes: [(new-transactions,[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349]), (new-block,[akka://blockchain/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349])], tombstones [] 11:05:44.904 [blockchain-akka.actor.default-dispatcher-15] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2035531976])] 11:05:44.904 [blockchain-akka.actor.default-dispatcher-17] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#1714452195])] 11:05:46.457 [blockchain-akka.actor.internal-dispatcher-20] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2553] - Change from replicator: [Map(ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block) -> Set(akka://blockchain/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480), ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions) -> Set(akka://blockchain/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480))], changes: [(new-transactions,[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewTransactions#-2092003166 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480]), (new-block,[akka://blockchain/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349, akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewBlock#-497024677 @ 5401420401358518049, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480])], tombstones [] 11:05:46.458 [blockchain-akka.actor.default-dispatcher-15] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#1714452195], Actor[akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewBlock#-497024677], Actor[akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180])] 11:05:46.458 [blockchain-akka.actor.default-dispatcher-17] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2035531976], Actor[akka://blockchain@127.0.0.1:2551/user/blockchainer/pubsubNewTransactions#-2092003166], Actor[akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065])] 11:05:47.367 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(69d4, 4500/2, 2021-05-11 18:05:47) to Blockchainer. 11:05:47.372 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: T(69d4, 4500/2, 2021-05-11 18:05:47) is published. 11:05:47.377 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(69d4, 4500/2, 2021-05-11 18:05:47) to transaction queue. 11:05:47.892 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(0060, 2000/1, 2021-05-11 18:05:47) to transaction queue. 11:05:48.285 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(bca5, 4000/3, 2021-05-11 18:05:48) to transaction queue. 11:05:53.552 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@5ef2baa2: BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567) is valid. Updating blockchain. 11:05:53.552 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:02.366 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(b137, 3500/2, 2021-05-11 18:06:02) to Blockchainer. 11:06:02.367 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: T(b137, 3500/2, 2021-05-11 18:06:02) is published. 11:06:02.368 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(b137, 3500/2, 2021-05-11 18:06:02) to transaction queue. 11:06:02.891 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(3b6c, 5500/2, 2021-05-11 18:06:02) to transaction queue. 11:06:03.289 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(36fa, 5500/3, 2021-05-11 18:06:03) to transaction queue. 11:06:12.467 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@5ef2baa2: BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119) is valid. Updating blockchain. 11:06:12.468 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:14.378 [blockchain-akka.actor.default-dispatcher-6] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 18000 millis 11:06:14.378 [blockchain-akka.actor.default-dispatcher-6] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:06:14.379 [blockchain-akka.actor.default-dispatcher-6] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(69d4, 4500/2, 2021-05-11 18:05:47), T(0060, 2000/1, 2021-05-11 18:05:47), T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03)) 11:06:14.380 [blockchain-akka.actor.default-dispatcher-6] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:06:17.030 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@5ef2baa2: BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357) is valid. Updating blockchain. 11:06:17.030 [blockchain-akka.actor.default-dispatcher-6] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:17.369 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(4919, 2500/2, 2021-05-11 18:06:17) to Blockchainer. 11:06:17.369 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: T(4919, 2500/2, 2021-05-11 18:06:17) is published. 11:06:17.370 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(4919, 2500/2, 2021-05-11 18:06:17) to transaction queue. 11:06:17.897 [blockchain-akka.actor.default-dispatcher-6] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(a892, 4500/2, 2021-05-11 18:06:17) to transaction queue. 11:06:18.291 [blockchain-akka.actor.default-dispatcher-3] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(45db, 2500/2, 2021-05-11 18:06:18) to transaction queue. 11:06:32.374 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(850c, 5000/3, 2021-05-11 18:06:32) to Blockchainer. 11:06:32.375 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: T(850c, 5000/3, 2021-05-11 18:06:32) is published. 11:06:32.376 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(850c, 5000/3, 2021-05-11 18:06:32) to transaction queue. 11:06:32.432 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 19000 millis 11:06:32.432 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:06:32.433 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(0060, 2000/1, 2021-05-11 18:05:47), T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03), T(4919, 2500/2, 2021-05-11 18:06:17), T(a892, 4500/2, 2021-05-11 18:06:17), T(45db, 2500/2, 2021-05-11 18:06:18), T(850c, 5000/3, 2021-05-11 18:06:32)) 11:06:32.434 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357), BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:06:32.902 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(bd5f, 4000/2, 2021-05-11 18:06:32) to transaction queue. 11:06:33.295 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(72cc, 4500/3, 2021-05-11 18:06:33) to transaction queue. 11:06:35.315 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@5ef2baa2: BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378) is valid. Updating blockchain. 11:06:35.316 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:37.926 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:06:37.928 [blockchain-akka.actor.default-dispatcher-18] WARN akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@5ef2baa2: BLK(7V0m, T(69d4, 4599/3), 2021-05-11 18:06:32, 3, 7774969) isn’t longer than existing blockchain! Blockchain not updated. 11:06:37.928 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:43.644 [blockchain-akka.actor.default-dispatcher-16] ERROR akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@5ef2baa2: ERROR: BLK(jy21, T(ac20, 2599/2), 2021-05-11 18:06:26, 3, 24589944) is invalid! 11:06:43.645 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:06:46.779 [blockchain-akka.actor.default-dispatcher-16] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2553] - Exiting confirmed [akka://blockchain@127.0.0.1:2551] <<< <<<-- Marked seed note #1 as `exiting confirmed` <<< 11:06:47.376 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(0e7e, 1000/1, 2021-05-11 18:06:47) to Blockchainer. 11:06:47.377 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: T(0e7e, 1000/1, 2021-05-11 18:06:47) is published. 11:06:47.378 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(0e7e, 1000/1, 2021-05-11 18:06:47) to transaction queue. 11:06:47.829 [blockchain-akka.actor.default-dispatcher-16] INFO akka.remote.artery.Association - Association to [akka://blockchain@127.0.0.1:2551] having UID [5401420401358518049] has been stopped. All messages to this UID will be delivered to dead letters. Reason: ActorSystem terminated 11:06:48.039 [blockchain-akka.actor.internal-dispatcher-2] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2553] - Node with registered services removed [UniqueAddress(akka://blockchain@127.0.0.1:2551,5401420401358518049)] 11:06:48.040 [blockchain-akka.actor.default-dispatcher-15] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2035531976], Actor[akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065])] 11:06:48.040 [blockchain-akka.actor.default-dispatcher-16] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#1714452195], Actor[akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180])] 11:06:48.298 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(3bfc, 2000/1, 2021-05-11 18:06:48) to transaction queue. 11:06:48.357 [blockchain-akka.actor.internal-dispatcher-2] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2553] - Change from replicator: [Map(ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-transactions) -> Set(akka://blockchain/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480), ServiceKey[akka.actor.typed.internal.pubsub.TopicImpl$Command](new-block) -> Set(akka://blockchain/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480))], changes: [(new-block,[akka://blockchain/user/blockchainer/pubsubNewBlock#1714452195 @ 5558056305511553349, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180 @ -3750460307660254480]), (new-transactions,[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2035531976 @ 5558056305511553349, akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065 @ -3750460307660254480])], tombstones [] 11:06:48.357 [blockchain-akka.actor.default-dispatcher-15] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewBlock#1714452195], Actor[akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewBlock#1064341180])] 11:06:48.358 [blockchain-akka.actor.default-dispatcher-16] DEBUG akka.actor.typed.pubsub.Topic$ - Topic list updated [Set(Actor[akka://blockchain/user/blockchainer/pubsubNewTransactions#-2035531976], Actor[akka://blockchain@127.0.0.1:2552/user/blockchainer/pubsubNewTransactions#-1565562065])] 11:06:51.488 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 15000 millis 11:06:51.488 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:06:51.489 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(bca5, 4000/3, 2021-05-11 18:05:48), T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03), T(4919, 2500/2, 2021-05-11 18:06:17), T(a892, 4500/2, 2021-05-11 18:06:17), T(45db, 2500/2, 2021-05-11 18:06:18), T(850c, 5000/3, 2021-05-11 18:06:32), T(bd5f, 4000/2, 2021-05-11 18:06:32), T(72cc, 4500/3, 2021-05-11 18:06:33), T(0e7e, 1000/1, 2021-05-11 18:06:47), T(3bfc, 2000/1, 2021-05-11 18:06:48) 11:06:51.490 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378), BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357), BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:06:59.436 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:06:59.438 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@5ef2baa2: BLK(AsCZ, T(0060, 2099/2), 2021-05-11 18:06:51, 3, 12440412) is valid. Updating blockchain. 11:06:59.438 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:07:02.382 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(130f, 4000/2, 2021-05-11 18:07:02) to Blockchainer. 11:07:02.383 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: T(130f, 4000/2, 2021-05-11 18:07:02) is published. 11:07:02.383 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(130f, 4000/2, 2021-05-11 18:07:02) to transaction queue. 11:07:03.304 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(6179, 4000/2, 2021-05-11 18:07:03) to transaction queue. 11:07:06.543 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 25000 millis 11:07:06.543 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:07:06.544 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(b137, 3500/2, 2021-05-11 18:06:02), T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03), T(4919, 2500/2, 2021-05-11 18:06:17), T(a892, 4500/2, 2021-05-11 18:06:17), T(45db, 2500/2, 2021-05-11 18:06:18), T(850c, 5000/3, 2021-05-11 18:06:32), T(bd5f, 4000/2, 2021-05-11 18:06:32), T(72cc, 4500/3, 2021-05-11 18:06:33), T(0e7e, 1000/1, 2021-05-11 18:06:47), T(3bfc, 2000/1, 2021-05-11 18:06:48), T(130f, 4000/2, 2021-05-11 18:07:02), T(6179, 4000/2, 2021-05-11 18:07:03)) 11:07:06.545 [blockchain-akka.actor.default-dispatcher-18] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(AsCZ, T(0060, 2099/2), 2021-05-11 18:06:51, 3, 12440412), BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378), BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357), BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:07:11.405 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Miner - [Mining] Miner.DoneMining received. 11:07:11.407 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@5ef2baa2: BLK(G7b0, T(bca5, 4099/4), 2021-05-11 18:07:06, 3, 7482632) is valid. Updating blockchain. 11:07:11.407 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:07:11.441 [blockchain-akka.actor.default-dispatcher-17] WARN akkablockchain.actor.Blockchainer - [Req.UpdateBlockchain] akkablockchain.actor.Blockchainer@5ef2baa2: BLK(WzL+, T(a81d, 7099/4), 2021-05-11 18:07:09, 3, 2734351) isn’t longer than existing blockchain! Blockchain not updated. 11:07:11.441 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.BlockInspector - [Validation] BlockInspector.DoneValidation received. 11:07:17.388 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(3ecc, 3000/2, 2021-05-11 18:07:17) to Blockchainer. 11:07:17.388 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: T(3ecc, 3000/2, 2021-05-11 18:07:17) is published. 11:07:17.389 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(3ecc, 3000/2, 2021-05-11 18:07:17) to transaction queue. 11:07:18.308 [blockchain-akka.actor.default-dispatcher-15] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(6d51, 3000/1, 2021-05-11 18:07:18) to transaction queue. 11:07:31.601 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Start mining in 18000 millis 11:07:31.601 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Getting transaction queue and blockchain ... 11:07:31.602 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Transaction queue: Queue(T(3b6c, 5500/2, 2021-05-11 18:06:02), T(36fa, 5500/3, 2021-05-11 18:06:03), T(4919, 2500/2, 2021-05-11 18:06:17), T(a892, 4500/2, 2021-05-11 18:06:17), T(45db, 2500/2, 2021-05-11 18:06:18), T(850c, 5000/3, 2021-05-11 18:06:32), T(bd5f, 4000/2, 2021-05-11 18:06:32), T(72cc, 4500/3, 2021-05-11 18:06:33), T(0e7e, 1000/1, 2021-05-11 18:06:47), T(3bfc, 2000/1, 2021-05-11 18:06:48), T(130f, 4000/2, 2021-05-11 18:07:02), T(6179, 4000/2, 2021-05-11 18:07:03), T(3ecc, 3000/2, 2021-05-11 18:07:17), T(6d51, 3000/1, 2021-05-11 18:07:18)) 11:07:31.603 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.Simulator - [MiningLoop] Blockchain: List(BLK(G7b0, T(bca5, 4099/4), 2021-05-11 18:07:06, 3, 7482632), BLK(AsCZ, T(0060, 2099/2), 2021-05-11 18:06:51, 3, 12440412), BLK(Y0IY, T(6e8e, 6599/4), 2021-05-11 18:06:31, 3, 5286378), BLK(J3qW, T(a545, 2599/2), 2021-05-11 18:06:14, 3, 3742357), BLK(4+uU, T(7634, 5099/4), 2021-05-11 18:05:59, 3, 19435119), BLK(en8Q, T(ac20, 2599/2), 2021-05-11 18:05:45, 3, 11826567), BLK(0T/f, T(5f17, 2099/2), 2021-05-11 18:05:39, 3, 2673242), BLK(p98k, T(fab1, 3099/2), 2021-05-11 18:05:13, 3, 2771128), BLK(kyHx, T(5c75, 4599/3), 2021-05-11 18:04:47, 3, 14448435), BLK(XF1C, T(----, 0/0), 1970-01-01 00:00:00, 0, 0)) 11:07:32.392 [blockchain-akka.actor.default-dispatcher-17] INFO akkablockchain.actor.TransactionFeeder - [TransactionFeeder] Submitting T(4684, 5000/2, 2021-05-11 18:07:32) to Blockchainer. 11:07:32.392 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Blockchainer - [Req.SubmitTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: T(4684, 5000/2, 2021-05-11 18:07:32) is published. 11:07:32.393 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(4684, 5000/2, 2021-05-11 18:07:32) to transaction queue. ^C <<< <<<--- User terminated program on the node (node #3) <<< [warn] Canceling execution... Cancelled: runMain akkablockchain.Main 2553 src/main/resources/keys/account2_public.pem [error] Cancelled: runMain akkablockchain.Main 2553 src/main/resources/keys/account2_public.pem [error] Use 'last' for the full log. 11:07:33.315 [blockchain-akka.actor.default-dispatcher-16] INFO akkablockchain.actor.Blockchainer - [Req.AddTransactions] akkablockchain.actor.Blockchainer@5ef2baa2: Appended T(f1fe, 1500/1, 2021-05-11 18:07:33) to transaction queue. 11:07:33.571 [blockchain-akka.actor.default-dispatcher-16] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2553] - Marked address [akka://blockchain@127.0.0.1:2553] as [Leaving] 11:07:33.924 [blockchain-akka.actor.default-dispatcher-16] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2553] - Exiting completed 11:07:33.926 [blockchain-akka.actor.default-dispatcher-16] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2553] - Shutting down... 11:07:33.927 [blockchain-akka.actor.default-dispatcher-16] INFO akka.cluster.Cluster - Cluster Node [akka://blockchain@127.0.0.1:2553] - Successfully shut down 11:07:33.930 [blockchain-akka.actor.internal-dispatcher-13] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - ClusterReceptionist [akka://blockchain@127.0.0.1:2553] - terminated/removed 11:07:33.934 [blockchain-akka.actor.internal-dispatcher-13] DEBUG akka.cluster.typed.internal.receptionist.ClusterReceptionist - Cancel all timers 11:07:33.938 [blockchain-akka.actor.default-dispatcher-33] INFO akka.remote.RemoteActorRefProvider$RemotingTerminator - Shutting down remote daemon. 11:07:33.940 [blockchain-akka.actor.default-dispatcher-33] INFO akka.remote.RemoteActorRefProvider$RemotingTerminator - Remote daemon shut down; proceeding with flushing remote transports. 11:07:34.970 [blockchain-akka.actor.default-dispatcher-33] WARN akka.stream.Materializer - [outbound connection to [akka://blockchain@127.0.0.1:2552], control stream] Upstream failed, cause: StreamTcpException: The connection has been aborted 11:07:34.970 [blockchain-akka.actor.default-dispatcher-33] WARN akka.stream.Materializer - [outbound connection to [akka://blockchain@127.0.0.1:2552], message stream] Upstream failed, cause: StreamTcpException: The connection has been aborted 11:07:34.990 [blockchain-akka.actor.default-dispatcher-33] INFO akka.remote.RemoteActorRefProvider$RemotingTerminator - Remoting shut down. |